Just a post to conclude the ST2 and ST3 code and to show how it should work.
Here is the how it works (animated gif needs to be supported by your browser; first seconds show ST without multi-row, then it is enabled):
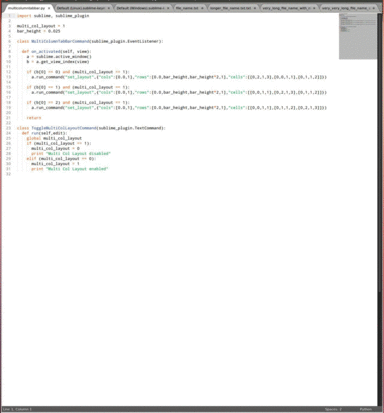
This is the plugin code for ST2
import sublime, sublime_plugin
multi_col_layout = 1
class MultiColumnTabBarCommand(sublime_plugin.EventListener):
def on_activated(self,view):
a=sublime.active_window()
b=a.get_view_index(view)
if (b[0] == 0) and (multi_col_layout == 1):
a.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, 0.025, 0.05, 1.0],"cells": [[0, 2, 1, 3],[0, 0, 1, 1],[0, 1, 1, 2]]})
if b[0] == 1 and (multi_col_layout == 1):
a.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, 0.025, 0.05, 1.0],"cells": [[0, 0, 1, 1],[0, 2, 1, 3],[0, 1, 1, 2]]})
if b[0] == 2 and (multi_col_layout == 1):
a.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, 0.025, 0.05, 1.0],"cells": [[0, 0, 1, 1],[0, 1, 1, 2], [0, 2, 1, 3]]})
class ToggleMultiColLayoutCommand(sublime_plugin.TextCommand):
def run(self,edit):
global multi_col_layout
if (multi_col_layout == 1):
multi_col_layout = 0
print "Multi Column switch disabled"
elif (multi_col_layout == 0):
multi_col_layout = 1
print "Multi Column switch enabled"
class DisableMultiColLayoutCommand(sublime_plugin.TextCommand):
def run(self,edit):
global multi_col_layout
multi_col_layout = 0
print "Multi Column switch disabled"
class EnableMultiColLayoutCommand(sublime_plugin.TextCommand):
def run(self,edit):
global multi_col_layout
multi_col_layout = 1
print "Multi Column switch enabled"
This is the plugin code for ST3
from collections import defaultdict
import sublime_plugin
enabled_map = defaultdict(lambda: True)
#modify this parameter to configure height of tab rows
bar_height = 0.025
class MultiColumnTabBarCommand(sublime_plugin.EventListener):
def on_activated(self, view):
id_ = view.window().id()
if not enabled_map[id_]:
return
window = view.window()
group, _ = window.get_view_index(view)
if group == 0:
window.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, bar_height, bar_height*2, 1.0],"cells": [[0, 2, 1, 3],[0, 0, 1, 1],[0, 1, 1, 2]]})
elif group == 1:
window.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, bar_height, bar_height*2, 1.0],"cells": [[0, 0, 1, 1],[0, 2, 1, 3],[0, 1, 1, 2]]})
elif group == 2:
window.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, bar_height, bar_height*2, 1.0],"cells": [[0, 0, 1, 1],[0, 1, 1, 2],[0, 2, 1, 3]]})
class ToggleMultiColLayoutCommand(sublime_plugin.WindowCommand):
def run(self):
id_ = self.window.id()
enabled_map[id_] ^= True
if enabled_map[id_]:
print("Multi Column switch enabled")
else:
print("Multi Column switch disabled")
class DisableMultiColLayoutCommand(sublime_plugin.WindowCommand):
def run(self):
id_ = self.window.id()
enabled_map[id_] = False
print("Multi Column switch disabled")
class EnableMultiColLayoutCommand(sublime_plugin.WindowCommand):
def run(self):
id_ = self.window.id()
enabled_map[id_] = True
print("Multi Column switch enabled")
class ResetLayoutCommand(sublime_plugin.WindowCommand):
def run(self):
id_ = self.window.id()
enabled_map[id_] = False
self.window.run_command("set_layout",{"cols": [0.0 ,1],"rows": [0.0, 1.0],"cells": [[0, 0, 1, 1]]})
print("Multi Column disabled and layout reset to standard")
And some keyboard shortcuts if you need them (ST2 tested only).
//layout: enable/disable multi line tabbar switching
{ "keys": "ctrl+u","ctrl+r"], "command": "toggle_multi_col_layout" },
//disable multiline tabbar and show single tabline only
{
"keys": "ctrl+alt+b"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "disable_multi_col_layout", "context": "window"},
{"command": "set_layout", "context": "window","args":{"cols": [0.0, 1.0],"rows": [0.0, 1.0],"cells": [0, 0, 1, 1]]}}
]}},
//split screen
{
"keys": "ctrl+alt+n"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "disable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0, 0.5, 1.0],
"rows": [0.0, 1.0],
"cells": [0, 0, 1, 1], [1, 0, 2, 1]]
}}
]}},
//move current file to other row
{ "keys": "ctrl+alt+,"], "command": "move_to_group", "args": { "group": 0 } },
{ "keys": "ctrl+alt+."], "command": "move_to_group", "args": { "group": 1 } },
{ "keys": "ctrl+alt+-"], "command": "move_to_group", "args": { "group": 2 } },
//first group to foreground
{
"keys": "ctrl+,"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "enable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 2, 1, 3],[0, 0, 1, 1],[0, 1, 1, 2]]
}}
]}},
//second group to foreground
{
"keys": "ctrl+."],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "enable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 0, 1, 1],[0, 2, 1, 3],[0, 1, 1, 2]]
}}
]}},
//third group to foreground
{
"keys": "ctrl+-"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "enable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 0, 1, 1],[0, 1, 1, 2], [0, 2, 1, 3]]
}}
]}},
//disable multi tabline view and show first row only
{
"keys": "ctrl+b"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "disable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 0, 1, 3],[0, 0, 0, 0],[0, 0, 0, 0]]
}}
]}},
//disable multi tabline view and show second row only
{
"keys": "ctrl+n"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "disable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 0, 0, 0],[0, 0, 1, 3],[0, 0, 0, 0]]
}}
]}},
//disable multi tabline view and show third row only
{
"keys": "ctrl+m"],
"command": "run_multiple_commands",
"args": {
"commands":
{"command": "disable_multi_col_layout", "context": "window"},
{
"command": "set_layout",
"context":"window",
"args":
{
"cols": [0.0 ,1],
"rows": [0.0, 0.025, 0.05, 1.0],
"cells": [0, 0, 0, 0],[0, 0, 0, 0],[0, 0, 1, 3]]
}}
]}}